Python is one of the powerful programming languages is a known fact to many of us. Even most of the surveys have reported that Python continues to remain as one of most popular programming language among the program developers. But the answer for a question that most of the tech enthusiasts wants to get is what are the facts that makes Python so powerful programming language?
There can be many answers for this question such as:
- It is one of the easiest programming language to learn
- It has simple syntax and follows a simple coding structure
- It provides large number of libraries for the developers.
Without a doubt that all the above points are the valid reasons why Python is considered a powerful programming language, but the one core aspect that needs to be mentioned here without which this programming language wouldn’t have reached this height and that is its extended support towards Object-Oriented concept.
Yes, you read it right! Python supports Object-Oriented Programming concept and is the biggest strength of this programming language. Object-Oriented programming is an approach to write the codes by creating Objects and Classes.
However, we found many aspiring Python developers are willing to learn this unique concept of programming and are search of right online courses on Python that enables them to learn. So, with an aim to help such people we are here presenting this blog that discusses about this concept of Python programming.
Before you start learning what is object oriented programming concept? At first we recommend you read this blog that discusses about some of the fundamental concepts of Python programming.
But if you are completely a new beginner to this Python world, then you start your Python learning curve with reading this blog that provides you a good introduction to Python programming. You can also watch this video that discuss about the same concept in detail.
One more important aspect of Python is: it not only supports Object-Oriented Programming Concept (OOP) but also supports Procedure Oriented Programming Concept (POP). Now you must be wondering what are the differences between OOP and POP, so to clear your doubt in this blog we are also discussing the differences between these two programming approaches.
In the upcoming discussion we will discuss the following concepts:
- Object-Oriented V/S Procedure Oriented Programming Concepts
- Object-Oriented Programming Concepts
- Python V/S Java
- Python V/S C++.
Now as mentioned above at first we will discus the differences between OOP and POP.
Object-Oriented V/S Procedure Oriented Programming Concepts
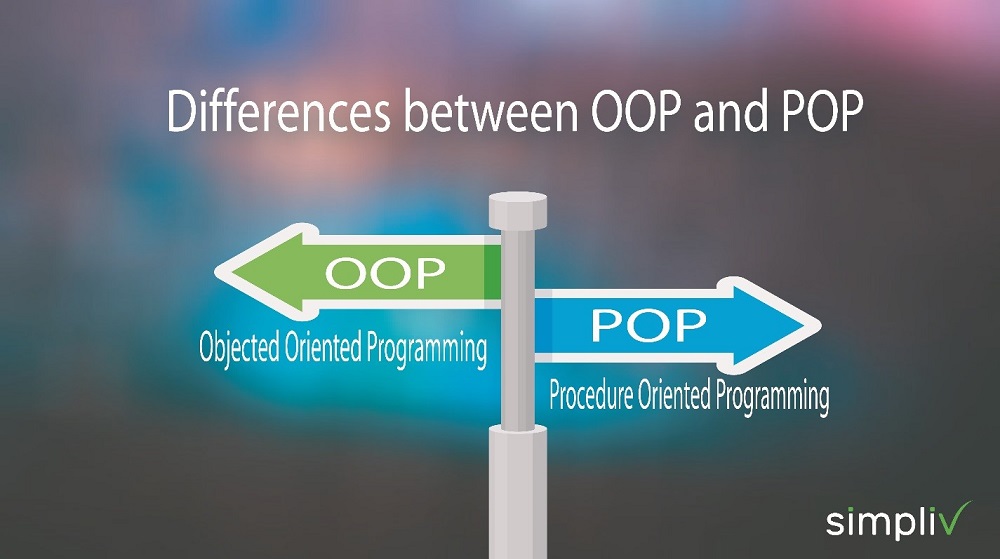
What is Object-Oriented programming concept?
Object-Oriented Programming is a concept that uses object and classes to create models based on real world environment. It is a specific way of designing a program. It can also be understood as an approach of writing a program that provides a means of structuring programs so that properties and behaviors are bundled into individual objects.
What is Procedural-Oriented programming concept?
Procedural-Oriented programming concept is conventional way of programming. It is a where prime focus is on getting the task done is sequential order.
Both programming approaches have certain advantages and disadvantages and developers needs to e aware of them while working on Projects using Python programming language.
Now let us discuss some of the differences that exist between these two programming approaches.
Sl.No. | Object-Oriented Programming Language | Procedure-Oriented Programming Language |
1 | OOP is a problem solving approach and it is used where computation is done using Objects, | POP uses a list of instructions to do computation step by step. |
2 | This approach uses classes. | This approach uses modules. |
3 | It support inheritance that allows to use the attributes and functions of the other class by inheriting it. | It doesn’t support inheritance. |
4 | It supports overloading/polymorphism. This enables to use the same function name for performing different functions. | It does not support the overloading/polymorphism. |
5 | It makes several access modifiers such as ‘public’, ‘private’, ‘protected’. | It does not support access modifiers. |
6 | Here program is divided into objects. | Here program is divided into functions. |
Object-Oriented Programming Concept
Now that we have understood some of the major differences between Object-Oriented Programming concept and Procedure Oriented Programming concept, now let us start discussing about Object-Oriented Programming concept.
Python Object Oriented Concept are as follows:
- Object
- Class
- Inheritance
- Polymorphism
- Data Abstraction
- Encapsulation
Class:
A class is a blueprint using which an object is created. It can be understood as a logical entity that helps defining in behavior and properties of an object. Classes are used to create a new user-defined data structures which contains arbitrary information about something.
For example, if you have an employee class then it should contain an attribute and method such an email Id, name, age etc.
You should know: Classes help the developers to reuse the code that makes the program more efficient. |
Syntax to declare Class:
A class in Python can be declared by using the class keyword, followed by the class name.
class ClassName: ‘optional class documentation string’ Class_suite |
Object:
Objects are the entity that has both state and behavior. It may be a real world object such as computer, chair, table etc. It is used to create an instance of the class. Everything in Python is an object and almost everything has attributes and methods.
Syntax of object:
obj = class1() |
The below program shows how to execute the class:
# example file for working with classes class myClass(): def method1(self): print(“online education platform”) def method2(self,someString): print(“Python:” + someString) def main(): # exercise the class methods c = myClass () c.method1() c.method2(“Python is easy to learn”) if __name__== “__main__”: main() |
The output of the above program will be as follows:
online education platform Python: Python is easy to learn |
Methods:
Methods are the functions that are defined inside the body of the class. These are used to define the behavior of the object. There are many method names that have special significance in Python classes.
Let us see the significance of __init__ method. This function is a reserved function is classes in Python. This is automatically called whenever a new object of the class is instantiated.
The below program shows how __init__ method works.
class Person: def __init__(self, name): self.name = name def say_hi(self): print(‘Hello, my name is’, self.name) p = Person(‘John’) p.say_hi() # The previous 2 lines can also be written as # Person(‘Swaroop’).say_hi() |
The output of the above program will be as follows:
Hello, my name is John |
Now let us start discussing about Inheritance concept of OOP.
Inheritance:
Inheritance is one of the important concept Object-Oriented Concepts. It is considered as one of the powerful feature. The ability of a class to inherit the properties of another class is known as inheritance.
Inheritance helps the developers to reuse the code. Here the developers can define a new class with less or no modification to an existing class. The new class is called derived (or child) class and one from where it inherits is called the base (or parent) class.
Inheritance allows to inherit attributes and methods from the base/parent class. This become very useful whenever there is a need to create subclasses and get all the functionality from the parent class.
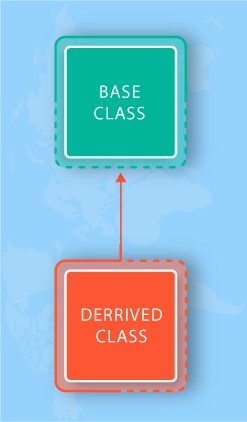
Syntax of Inheritance:
class derived_Class_Name (base class): |
The below program shows the example of Python inheritance example:
#Program example to show Python inheritance #Parent class is created class onlinecourses: def function1(self): print(“Online learning platfrom”) #Child class is created class program(onlinecourses): def function2(self): print(“Python program”) ob = program() ob.function1() ob.function2() |
The output of the above program will be as follows:
Online learning platform Python program |
There are different types of Inheritance available in Python and they are as follows:
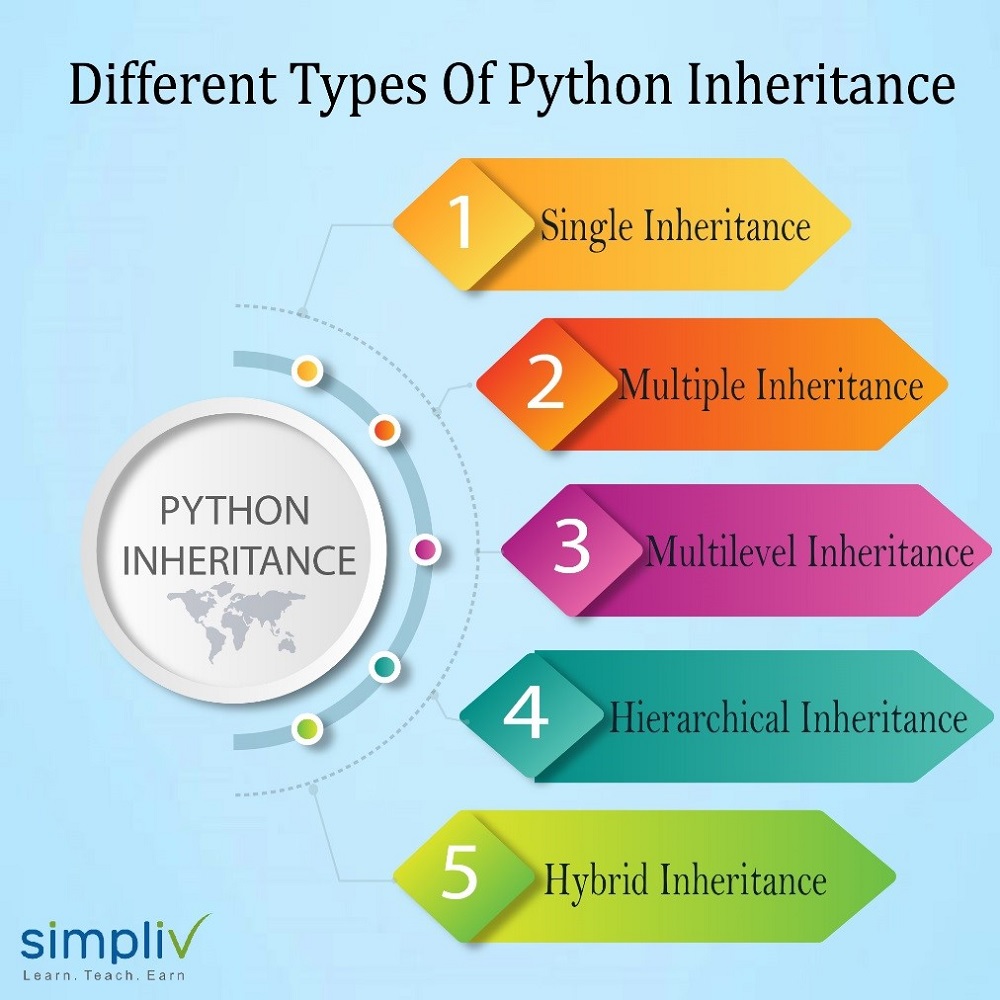
Let us discuss each one of them separately.
- Single inheritance:This is a type of inheritance in which a class inherits only one base class.
The below program shows the example for single inheritance.
#The programming example to show single inheritancee #Parent class is created class Parent: def function1(self): print(“This is first function”) # Child class is created class Child(Parent): def function2(self): print(“This is second function”) ob = Child() ob.function1() ob.function2() |
The output of the above program will be as follows:
This is first function This is second function |
- Multiple Inheritance:
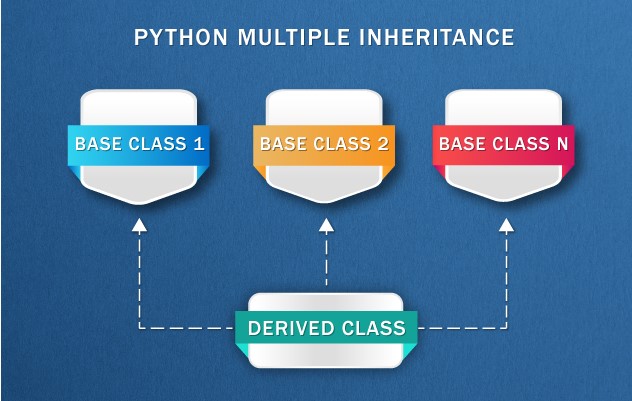
Python allows the developers to derive a class from several classes at once, which is known as Multiple Inheritance. In this type of inheritance, a class inherits multiple base classes.
The below program shows the example for multiple inheritance
# The programming example to show Multiple Inheritance # Parent class is created class Parent: def function1(self): print(“This prints function1”) class Parent2: def function2(self): print(“This prints function2”) # Child class is created class Child(Parent , Parent2): def function3(self): print(“This prints function3”) ob = Child() ob.function1() ob.function2() ob.function3() |
The output of the above program will be as follows:
This prints function1 This prints function2 This prints function3 |
- Multilevel Inheritance:
In this type of inheritance, a class inherits a base class and then another class inherits this previously derived class, forming a ‘parent, child, and grandchild’ class structure, then it’s known amultilevel inheritance.
The following program will show how Multilevel Inheritance will work in Python
class Onlinecourses: def function1(self): print(“Online learning platform”) class Pythoncourses(Onlinecourses): def function2(self): print(“Python learning courses”) class Pythonforbeginners(Pythoncourses): def function3(self): print(“This is Python for beginners course”) ob = Pythonforbeginners() ob.function1() ob.function2() ob.function3() |
The output of the above program will be as follows:
Online learning platfrom Python learning courses This is Python for beginners course |
- Hierarchical Inheritance:
In this type of inheritance only one base class is inherited from multiple derived classes. Here it involves multiple inheritance from the same base or parent class. It can be understood as when more than one class is derived from the single base class, such inheritance can be called as Hierarchical Inheritance.
The below program shows the example of Hierarchical Inheritance.
class Parent: def function1(self): print(“This prints function 1”) class Child(Parent): def function2(self): print(“This prints function 2”) class Child2(Parent): def function3(self): print(“This prints function 3”) ob = Child() ob1 = Child2() ob.function1() ob.function2() |
The output of the above program is as follows:
This prints function 1 This prints function 2 |
- Hybrid Inheritance:
Hybrid Inheritance is a combination of several inheritance. It combines more than of one form of inheritance.
The below program will show how Hybrid Inheritance works in Python
class classA:
def function1(self): print(“This is class A method”) class classB(classA): def function2(self): print(“This is class B method”) class classC(classA): def function3(self): print(“This ic class C method”) class classD(classB,classC): def function4(self): print(“This ic class D method”) obj=classD() obj.function1() obj.function2() obj.function3() obj.function4() |
The output of the above program will be as follows:
This is class A method This is class B method This is class C method This is class D method |
Now that we have understood how Inheritance works in Python now let us start discussing yet another important concept of Python programming i.e. Polymorphism.
Polymorphism
Polymorphism means one task can be performed in multiple ways. In Object-Oriented Programming Concept Polymorphism allows the developers to define methods in the child class with the same name as defined in their parent class.
As is already known,child class inherits all the methods from the parent class, however, in some cases the inherited method doesn’t exactly fit into the child class. So, in such situations to runtime errors, developers consider the option to re-implement method in the child class and this process is called as Method overriding.
Now suppose a method has been overridden in the child class, then the new version of the method needs to be called on basis of object type that is used for invoking the same.
The version of the method will be called as follows:
- In cases where a child class object has been used for calling an overridden method, the child class version belonging to the method will be called.
- In cases where a parent class object has been used for calling an overridden method, the parent class version belonging to the method will be called.
Polymorphism is very useful for Python developers as it makes coding very easier.
Encapsulation:
Encapsulation is one of the important concept of Object-Oriented Programming. It gives developer more control over the degree of coupling in their code. It allows a class to change its implementation without affecting other parts of the code.
Data Abstraction:
Abstraction means hiding the complexity and show only functionalities. In Python Abstraction is achieved by using abstract classes and interfaces.
Data Abstraction refers to providing the only the essential information about the data to the external world and thus hides the implementation. Abstraction are used in the programming languages to make abstract class.
5 Advantages of using Object-Oriented Programming Concept
Now as we have discussed about different Object-Oriented Programing Concept, now let us understanding the some of the advantages of it.
- Code reusability: It is one of the characteristics of OOP. Code reusability means reusing the same code again if similar features or functionality is required. This feature saves a lot of time while developing projects.
- Easy to maintain code: Maintain of code becomes very easy in OOP. It is always easy and time-saving to maintain and modify the existing codes as new objects are created with small differences to existing ones.
- Faster development:As parallel development of classes is possible in OOP concept; it results in quick development of projects.
- Reduced development cost: As the objects created for Object-Oriented programs can be reused in other program, it saves huge amount development cost.
- Secured:In OOP since the data is hidden and cant be accessed by external functions itisconsidered to be secured development technique.
Python V/S Java
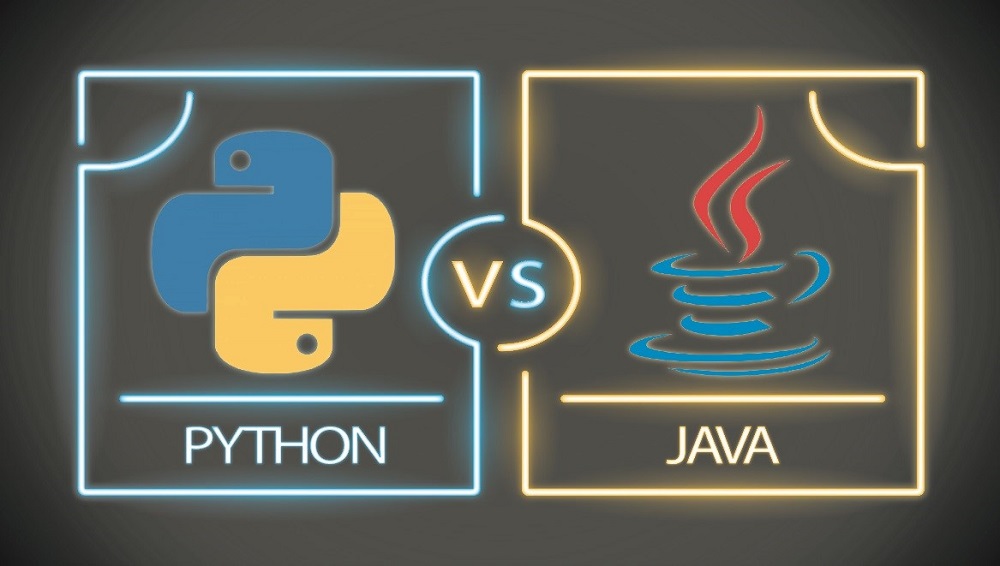
Python and Java both are the popular programming languages. These two languages have been widely used by many programmers while developing the software application. Let us see some of the difference that exists between these two programming languages.
Comparison factor | Python | Java |
Definition | Python is a high-level, Objective-Oriented programming language that is mostly used to develop applications such as web development, Artificial Intelligence, Machine Learning etc. | Java is a general-purpose, Object-oriented programming language that is mainly used to develop applications such as mobile applications, enterprise applications etc. |
Code |
Shorter lines of codes in comparison to Java Ex: print (“Hello world”) |
Longer lines of code are written in Java Ex: Public class Program { Public static void main(String[]args) { System.out.println(“Hello world”); } } |
Speed | In terms of speed Python is slower. It is an interpreted programming language and determines the type of data at run time which makes it slower comparatively. | Java is comparatively faster than Python. |
Multi-threading | Python uses Global Interpreter Lock(GIL). It allows only a single thread to run at a time. | Java is multi-threading can support two or more threads which runs at the same time. |
Number of classes | In Python any number of classes can exists in a single file in Python. | In Java only one top-level class can exist in a single file in Java. |
Platform dependencies | Python is platform dependent programming language. | Java is a platform independent programming language. |
Python V/S C++
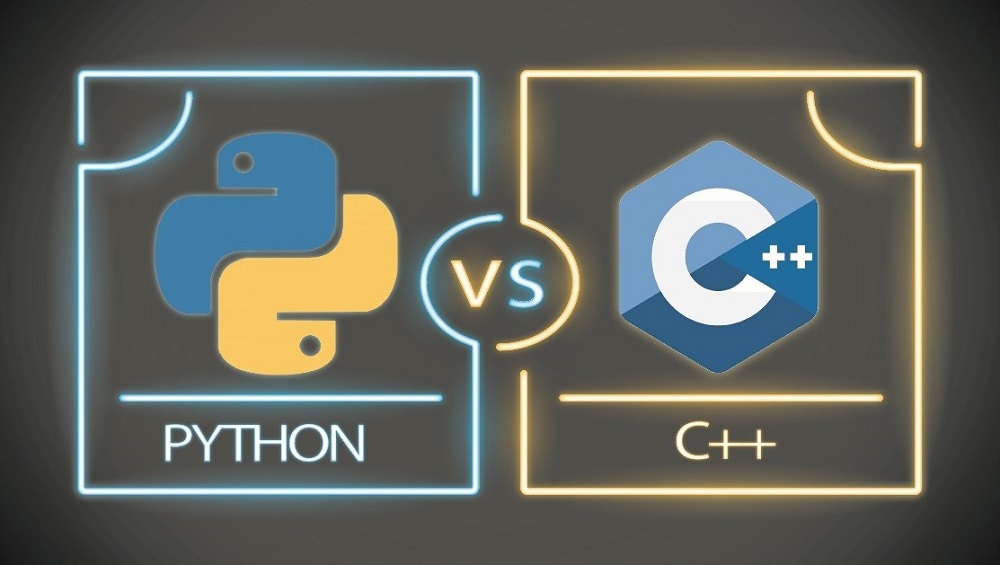
Python and C++ programming languages are very different in terms of their syntax, use and overall approach of programming. These two languages are very popular among the developers and they are being used while building different kinds of applications. Python is highly preferred of Artificial Intelligence and Machine Learning projects, while C++ is best option when it comes to game development.
Now let us discuss some of the differences that exists between Python and C++.
Comparison factor | Python | C++ |
Definition | Python is an interpreted, Objected-Oriented, high-level programming language. It is easy to write the code in Python as it has simple syntax. | C++ is general-purpose, Objected-Oriented programming language. In comparison to Python, it is not easy to write a code in C++ due to complex syntax. |
Compilation | Python runs through interpreter. | C++ uses compiler for the compilation of the code. |
Nature | It is dynamically typed programming language. | It is Statistically typed programming language. |
Garbage Collection | Python supports Garbage Collection. | C++ does not support Garbage Collection |
Speed | In terms of speed Python is comparatively slower. | In comparison to Python C++ is faster. |
Scope of the variables | In Python, variables can be accessed even outside the loops. | In C++, the scope of variables limited within the loops. |
Conclusion:
Object-Oriented Programming concept is one of the important factor of Python programming. This feature provides a lot of flexibility to Python developers while working on software projects using this programming language.
This approach of program allows developers several benefits like code reusability, saves a lot of time, reduce the cost of development of projects etc. We hope that our readers have found some valuable information reading this blog.
Do you have any questions with regards to above discussed topics, please feel free to share them with us in the comment section. And if you think some more new concepts needed to be discussed here, then please send your feedback in the comment section.
We will be discussing about some more new concepts about this programming in our next blog.
With all the information above, now at this point we would like to introduce you to these online courses on Python provided Simpliv. We hope that you find them very valuable and guide to become a master in this programming language.
If you think this blog has helped to upscale your Python knowledge, then we request you to please share it among your circles so that it reach to someone who is looking for similar kind of reading.
Key Takeways:

- Python supports both Object-Oriented Programming Concept as well as Procedural-Oriented Programming Concept.
- Class is a logical entity that helps in defining both behavior and properties of an Object.
- Object-Oriented programming concept helps code reusability. It means same code can be used if similar feature or function is required.
- Inheritance is one of important feature of OOP. It is the ability of class to inherit the properties of another class.
- Polymorphism is OOP feature that allows the developers to define the methods in the child class with the same name as defined in their parent class.